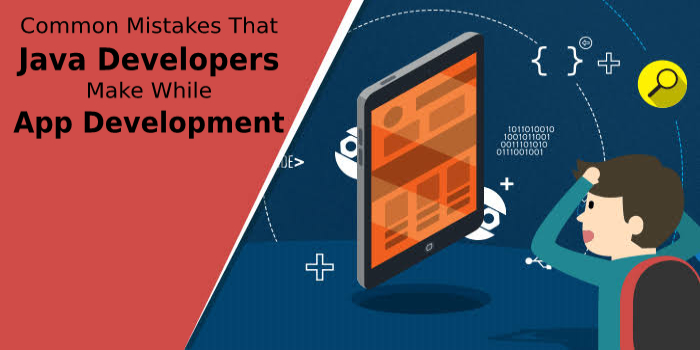
5 Common Mistakes That Java Developers Make While App Development
Java is a reputed programming language serving business enterprises from the last two decades. Designed with object-oriented programming features, eliminating the complexities of other languages including C or C++, garbage collection, and a virtual machine. Java created a different way of programming and follows “Write once, run everywhere” concept. This has completely changed the web application development process, but Java problems are still present. In this post, a few common mistakes are discussed that developers usually commit while development. These mistakes if addressed properly can boost the Java web development company productivity to a great extent.
1. Ignoring Existing Libraries
It’s definitely a huge mistake for developers to ignore the vast collection of libraries written in Java. Before writing any code, it is important to search for available libraries, several of them have been improved over the years and are free to use. Different sorts of logging libraries, like logback and Log4j, and network related libraries, like Netty or Akka are available to ease the development work.
2. Wrong Switch-Case Syntax
This Java issue is very embarrassing for the developers and sometimes remain hidden until executed. Fallthrough behavior present in switch statements is highly useful, but missing the “break” keyword in the syntax can lead to disastrous results. If “break” is forgotten in “case 0” as mentioned below, the code will execute “Zero” followed by “One”. The control flows present inside will compile through the whole “switch” statement until “break” appears. For example:
public static void switchCasePrimer() {
int caseIndex = 0;
switch (caseIndex) {
case 0:
System.out.println("Zero");
case 1:
System.out.println("One");
break;
case 2:
System.out.println("Two");
break;
default:
System.out.println("Default");
}
}
Such mistakes can be identified using the static code analyzers and the perfect solution is to use polymorphism and move code into separate classes.
3. Keeping Resources Engaged
Every time a program uses a file or network connection, it is highly crucial for the Java developers to free the resources once utilized. Take necessary measures if any exception were to be made during operations on those resources. You might be thinking that the FileInputStream has a finalizer that blocks the close() method on the garbage collection event, but this can’t be assured. It has been noticed that when a garbage collection starts, the input stream can access the computer resources for an indefinite period of time. Java development services deal with this problem, by implementing a try-with-resources statement to streamline the application development work.
private static void printFileJava7() throws IOException {
try(FileInputStream input = new FileInputStream("file.txt")) {
int data = input.read();
while(data != -1){
System.out.print((char) data);
data = input.read();
}
}
}
4. Memory Leaks
Java implements automatic memory management and is a big relief to allocate and free memory manually. But this doesn’t mean that Java developer should not be aware of memory management in the application. Problems with memory allocations usually happen and as long as the program creates references to objects which are useless, it will not be freed. This results in a memory leak and it can happen in multiple ways, but the major reason is everlasting object references. The garbage collector can’t clear objects while there are still references to them. Another possible reason for memory leaks is a group of objects referencing each other, leading to circular dependencies.
A common example of a memory leak could look like the following:
final ScheduledExecutorService scheduledExecutorService = Executors.newScheduledThreadPool(1);
final Deque numbers = new LinkedBlockingDeque<>();
final BigDecimal divisor = new BigDecimal(51);
scheduledExecutorService.scheduleAtFixedRate(() -> {
BigDecimal number = numbers.peekLast();
if (number != null && number.remainder(divisor).byteValue() == 0) {
System.out.println("Number: " + number);
System.out.println("Deque size: " + numbers.size());
}
}, 10, 10, TimeUnit.MILLISECONDS);
scheduledExecutorService.scheduleAtFixedRate(() -> {
numbers.add(new BigDecimal(System.currentTimeMillis()));
}, 10, 10, TimeUnit.MILLISECONDS);
try {
scheduledExecutorService.awaitTermination(1, TimeUnit.DAYS);
} catch (InterruptedException e) {
e.printStackTrace();
}
Also Read: Top 5 Advanced Features Expected In Java 14 From Oracle
5. Ignoring Exceptions
It is always soothing to leave exceptions unhandled. But the best practice for naive and experienced Java developers is to handle them. Exceptions are thrown on purpose, hence in most scenarios, we need to address the problems causing these exceptions. Do not overlook these, if necessary, you can simply rethrow it, show an error dialog to the user, or simply add a message to the log. At the very least, it should be explained why the exception has not been handled in order to let other developers know the reason.
selfie = person.shootASelfie();
try {
selfie.show();
} catch (NullPointerException e) {
}
A better approach of highlighting the exceptions’ insignificance is to encode this message into the exceptions’ variable name, like this:
try { selfie.delete(); } catch (NullPointerException unimportant) { }
Frequently Asked Questions
1. What are the errors in Java?
An Error “refers to serious problems that an application can’t catch.” Both Errors and Exceptions are the subclasses of java.lang.Throwable class. Errors are the situations which are difficult to recover by any handling techniques. It surely causes program termination in an abnormal manner.
2. What are the three types of errors in Java?
There are three types of errors: syntax errors, runtime errors, and logic errors.
3. Why is Java so important?
Java is a renowned programming language used to create Web and mobile applications. It was designed for flexibility and scalability, allowing developers to write code that has a feature to run on any machine, regardless of the architecture or platform.
4. Why Java is Object-Oriented?
Java is purely object-oriented programming language because without class and object it is impossible to write any Java program. Because Java supports non-primitive data types like int, float, boolean, double, long, etc.
Wrapping Up
Java as a platform simplifies multiple things in software development, depending on JVM and the language itself. The features like removing manual memory management don’t fix all the problems and issues, it is important to hire Java developer having expertise in building secure & engaging web application. In addition to that knowledge, practice and Java tutorials are available to avoid and address application errors. Don’t forget to use static code analyzers, as they point to the real bugs.